 |
Lecture 9: Loops I: for
|
|
Loops are for repeating part of the code a number of times. In C there
exist three types of loops,
There is no difference between the first two types of loops, only a
matter
of legibility of the program. The do .. while loop differs from the
other
two by the fact that the condition of exiting the loop is checked at
the
end, while for the other two (for and while) it is checked in the
beginning
of the loop.
Todays lecture is about the for loop.
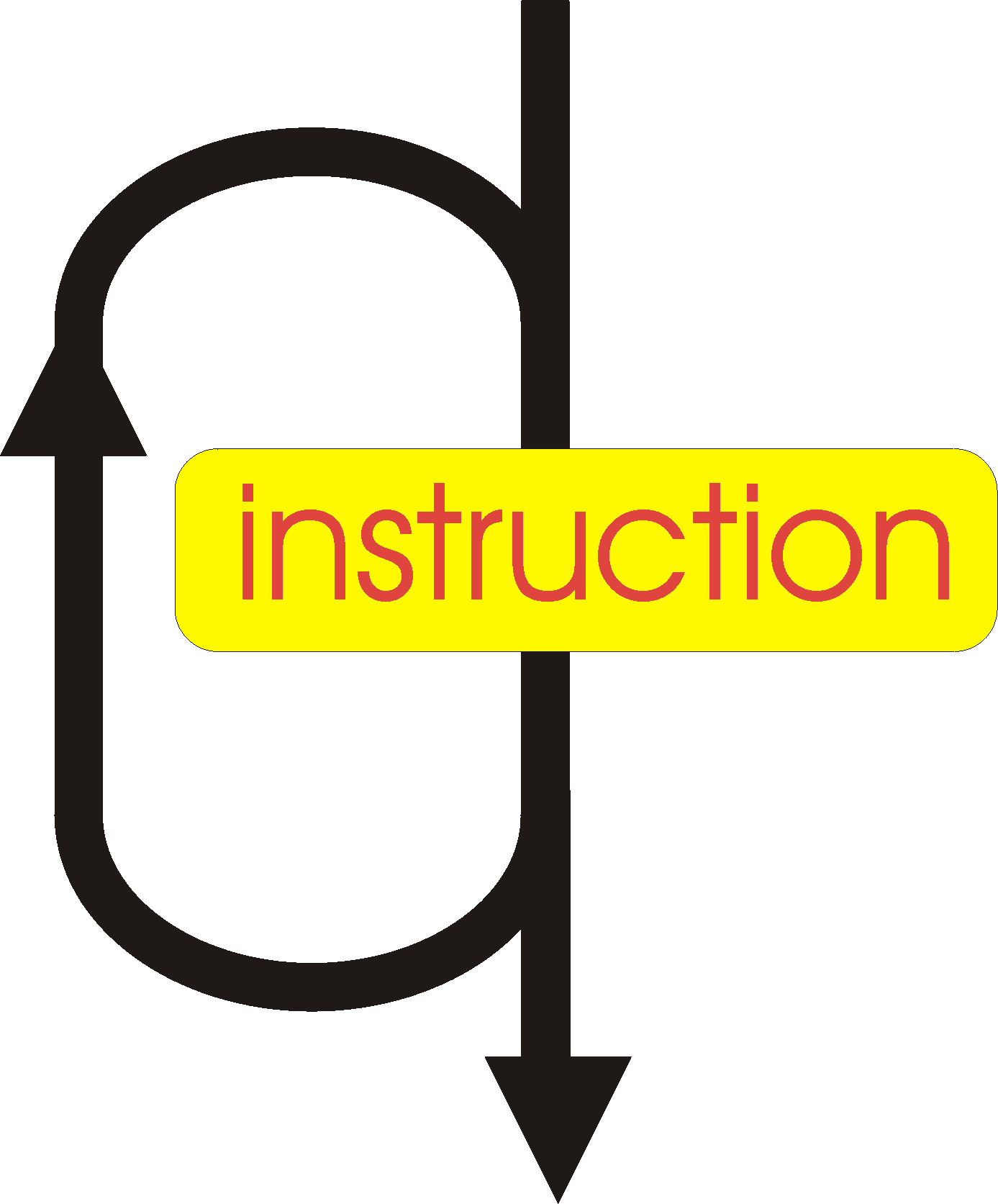 |
For loop
The most common loop in C is the for loop. This loop, in principle, is
used to execute things a predetermined number of times in a countable
way. This in contrast to loops that will run while a certain condition
is true, as we will learn in the next lecture. |
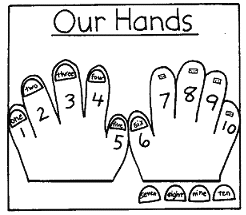 |
The general structure of the for loop is
for (startI;
testC;
stepI)
instruction; |
with startI and stepI
general instructions and testC a
Boolean condition. This will repeat the instructions instruction
and stepI until the test
condition
results in "false".
The
instruction
is repeated a number of times, determined by the control instructions startI
and stepI and the test condition
testC.
The control instructions startI
and
stepI
can be any instruction or even combination of instructions (separated
by
commas).
The startI instruction is only
executed at the beginning of the loop and only executed once.
Immediately
after that, the condition testC
is evaluated. If this results is "false" (0), the loop is immediately
exited.
Hence
it is possible with the for loop that the instruction
is not even executed a single time (contrary to the do ..
while
loop as we will see in the next lecture). If the result of the
condition
is "true" (!=0), the instructions instruction
and stepI are executed. |
Since the loop is designed for doing things in a countable way,
it is advised to use control variables of the integer type as in the
next
example. Do not forget to declare the variable (see lecture
5).
program code
main()
// for-loop example
{
int i;
for (i=1; i<5;
i++)
printf("Ola\n");
}
|
output
Ola
Ola
Ola
Ola |
This program is doing the following
1) it is attributing 1 to i
2) it is checking if i is smaller than 5
3) if not so: EXIT LOOP immediately. Else
4) execute printf("Ola\n");
5) add 1 to i
6) go to step 2)
|
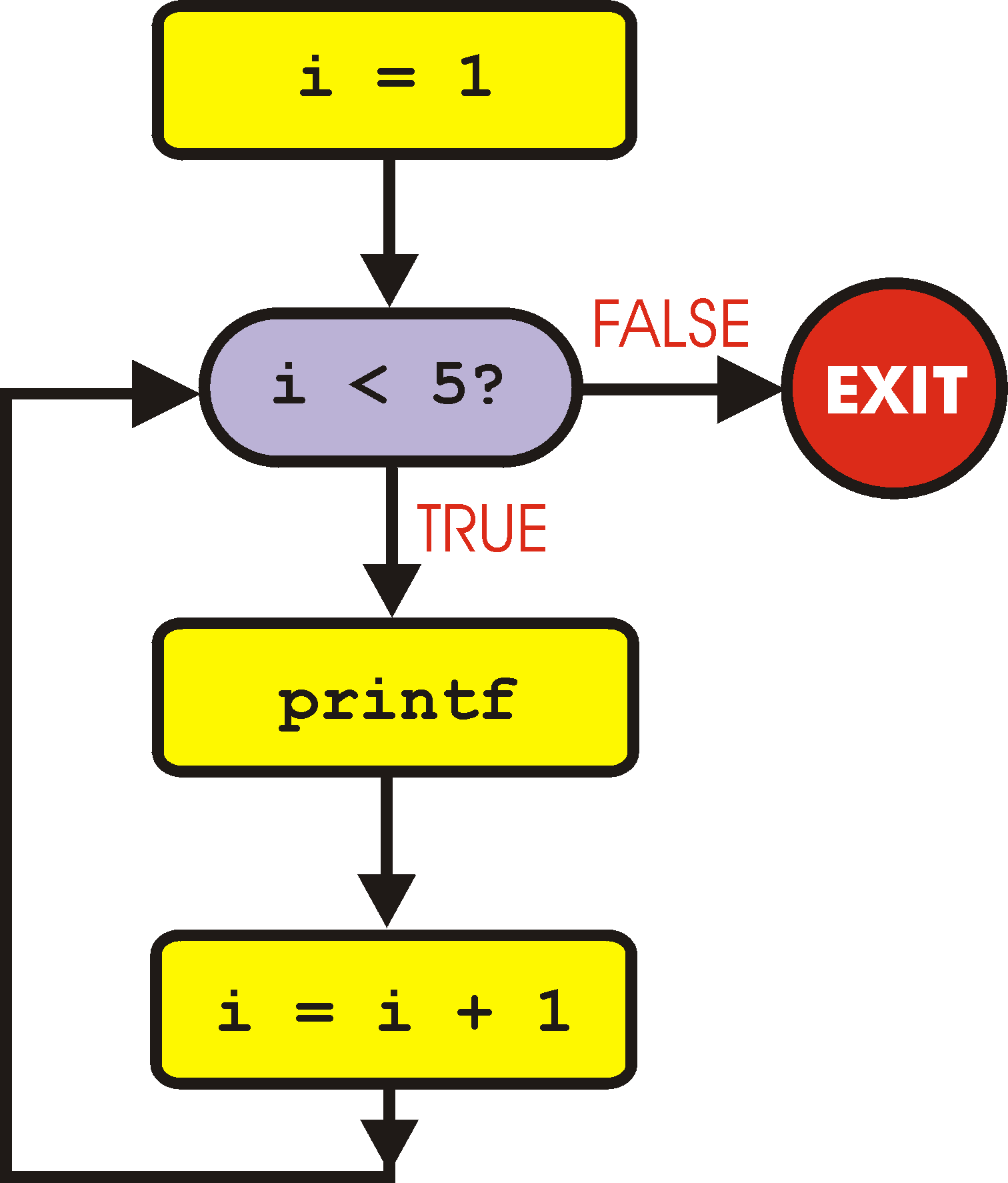 |
Multiple instructions
Just like with the if ... else ... structure, we
can also group instructions together with {..} in
loops:
program code
for (i = 1; i<5;
i++)
{
printf("Ola\n");
printf("It is a nice
day\n");
}
|
output
Ola
It is a nice day
Ola
It is a nice day
Ola
It is a nice day
Ola
It is a nice day
|
Compare this with
program code
for (i = 1; i<5;
i++)
printf("Ola\n");
printf("It is a nice day\n");
|
output
Ola
Ola
Ola
Ola
It is a nice day
|
Use of the loop variable
Inside the loop the loop variable can be used, but don't
mess with it
Good code:
program code
for (i=1; i<5; i++)
printf("%d Ola\n", i);
|
output
1 Ola
2 Ola
3 Ola
4 Ola
|
|
Bad code:
program code
for (i=1; i<5; i++)
{
printf("%d
Ola\n", i);
i =
i + 1;
}
|
output
1 Ola
3 Ola
|
|
The program on the right is an example of bad code. Such style of
programming,
although at some times it will save space and maybe executing time,
makes
your program unstructured and very difficult to understand for others!
If you want to achieve things like in the program on the right, use
other
loops, like while or do-while, or , better, use
something
like in the program below.
program code
for (i=1; i<5; i++)
printf("%d Ola", 2*i-1); |
output
1 Ola
3 Ola |
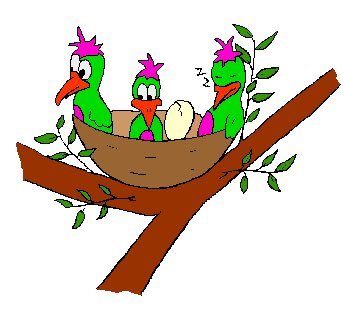 |
Nested loops
The For loops (and any other loop as well) can also be 'nested',
which means that they can be put within eachother. We can create double
loops, or triple loops (like in the figure below on the left) or any
other
level. Such structures look like nests of birds and hence the name 'nesting'
of loops. Here are some examples
|
|
|
program
code
--------------------------------------
main()
// three nested
loops
{
int i, j, k;
for
(i=1; i<=2; i++)
for
(j=1; j<=2;
j++)
for
(k=1; k<=2; k++)
printf("i=%d j=%d k=%d\n", i, j, k);
}
|
output
-------------
i=1 j=1 k=1
i=1 j=1 k=2
i=1 j=2 k=1
i=1 j=2 k=2
i=2 j=1 k=1
i=2 j=1 k=2
i=2 j=2 k=1
i=2 j=2 k=2 |
|
program code
------------------------------------------
main()
// three nested
loops
{
int i, j, k;
for
(i=1; i<=2; i++)
for
(j=1; j<=2;
j++)
{
for (k=1; k<=2; k++)
printf("i=%d j=%d k=%d\n", i, j, k);
for (k=1; k<=2; k++)
printf("i=%d j=%d k=%d\n", i, j, k);
}
}
|
output
-------------
i=1 j=1 k=1
i=1 j=1 k=2
i=1 j=1 k=1
i=1 j=1 k=2
i=1 j=2 k=1
i=1 j=2 k=2
i=1 j=2 k=1
i=1 j=2 k=2
i=2 j=1 k=1
i=2 j=1 k=2
i=2 j=1 k=1
i=2 j=1 k=2
i=2 j=2 k=1
i=2 j=2 k=2
i=2 j=2 k=1
i=2 j=2 k=2 |
|
An example. Fibonacci.
Fibonacci numbers are numbers that follow the following
rules:
F1 =1
F2 = 1
Fn = Fn-1 + Fn-2 |
|
The program below implements this. The user is asked to give the
number
of Fibonacci numbers to calculate (n) and then, in a for-loop, the next
Fibonacci number is calculated, until there are enough numbers.
main()
/* program to calculate the first n Fibonacci
numbers
using the algorithm
F(1) = 1, F(2) = 1 and F(i)
= F(i-1) + F(i-2) */
{
int i, n, fi, fi1, fi2;
// first ask the user for the
number
of Fibonacci numbers to calculate
printf("How many Fibonacci numbers
do you want to see?\n");
scanf("%d", &n);
fi1 =
1;
// variables to store F(i-1) and F(i-2)
fi2 =
1;
// initialize them to their starting value
printf("1 1
");
// print the first 2 Fibonacci numbers
// we are going to do something in
a countable way, so we will use
// the for-loop structure:
for (i=3; i<=n; i++)
// print the rest
{
fi = fi1 +
fi2; // calculate the next number
fi2 =
fi1;
// calculate the new F(i-1) and F(i-2)
fi1 = fi;
printf("%d
", fi); // print the result
}
}
Later we will learn a much more elegant way to calculate Fibonacci
numbers using recursive programming.
Quick test:
To test your knowledge of what you have learned in this lesson, click here
for an on-line test.
Peter Stallinga. Universidade do Algarve, 30
October
2002