 |
Lecture 13: Modular Programming II:
Procedures with input and output
|
|
In the previous lecture (aula 12) we have seen
Procedures that are not accepting parameters and are not generrating
return
values. These are simple procedures. Now we are going to look at
Procedures
that are accepting parameters and Procedures that are producing return
values (Functions).
Parameters to pass to Procedures
We can pass parameters to procedures. The procedure can then work with
these parameters. In PASCAL the parameters the procedure expects are
put
after the name of the procedure inside parenthesis:
Procedure ProcedureName(parameter_list);
Var <variable_list>;
Const <const_list>;
begin
instructions;
end;
|
|
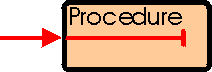 |
The variables on the parameter list are declared in the same way as
the normal variables of a program or procedure, namely we have to
specify
the type of the variable. Inside the procedure we can use the parameter
as iff it were a normal variable. We can calculate with it, use it in
conditions,
and even change its value.
As an example, the following program will calculate and show the
square
of a variable x: Note the way the parameter r is
declared
and used.
program code
PROGRAM WithParameters;
Var x: real;
PROCEDURE WriteSquare(r: real);
var y: real;
begin
r := r*r;
y := r;
Writeln('The suare of ',r:0:1,'
is
',y:0:1);
end;
begin
x := 4;
WriteSquare(x);
WriteSquare(3.0);
end.
|
output
The square of 4.0 is 16.0
The square of 3.0 is 9.0 |
As seen in the program above, the procedure with parameters can now
be called with a variable, as in WriteSquare(x)
or with a constant.as in WriteSquare(3.0).
Another example, that uses two parameters:
program code
PROGRAM WithParameters;
Var x: integer;
y: integer;
PROCEDURE WriteSum(i1, i2: integer);
( will write the sum of i1 and
i2
*)
var j: integer;
begin
j := i1+i2;
Writeln('The sum of ',i1,' and ',
i2, ' is ', j);
end;
begin
x := 4;
y := 5;
WriteSum(x, y);
WriteSum(3, 4);
end.
|
output
The sum of 4 and 5 is 9
The sum of 3 and 4 is |
Finally, an example with a parameter list of mixed types. Just like
in normal variable decalration, variables to be declared in the
parameter
list are separated by ;
program code
PROGRAM WithParameters;
PROCEDURE WriteNTimes(r: real; n:
integer);
(* Will write n times the real r
*)
var i: integer;
begin
for i := 1 to n do
WriteLn(r:0:3);
end;
PROCEDURE WriteFormatted(r: real; n:
integer);
(* Will write the real r with n
decimal
cases *)
begin
WriteLn(r:0:n);
end;
begin
WriteNTimes(3.0, 4);
WriteFormatted(5.0, 5);
WriteFormatted(5.0, 1);
end.
|
output
3.000
3.000
3.000
3.000
5.00000
5.0 |
Functions
Functions are procedures that are returning an output value. The type
of the returning value has to be specified at the moment of declaring
the
function, after the parameterlist (if any), preceded by a colon :
Example:
Function FunctionName(parameter_list):
type;
Var <variable_list>;
Const <const_list>;
begin
instructions;
end;
|
|
Functions without and with input
parameters
|
Somewhere in the instructions we have to specify a returning
value
by.
For example
FUNCTION Square(r: real): real;
(* will return the square
of of the parameter r *)
begin
r := r*r;
Square := r;
end;
At the place where the function will be called, we can assign this
value
to a variable (of the same type as the returning value of the
function!),
for example
y := Square(3.0);
use it as part of an expression, for example
y := 4.0 * Square(3.0) + 1.0;
or use it in another function or procedure, for example
Writeln(Square(3.0):0:1);
A full example:
program code
PROGRAM WithParameters;
Var x, y: real;
FUNCTION Square(r: real): real;
(* will return the square of of
the
parameter r *)
begin
r := r*r;
Square := r;
end;
begin
x := 4.0;
y := Square(x);
WriteLn('The square of ', x:0:1,
'
is ', y:0:1);
WriteLn('The square of ',
3.0:0:1,
' is ',
Square(3.0):0:1);
end.
|
output
The square of 4.0 is 16.0
The square of 3.0 is 9.0 |
Why?
Now the big question is "why?". Why write procedures and functions if
we
can do the same thing with normal lines of instructions? Indeed, the
first
languages (for example BASIC) didn't have the possibility to write
functions
and still we could write programs to solve any problem with it. There
are
however two important reasons why to use modules.
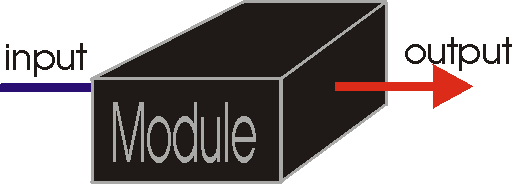
-
With modules, because they are like black boxes, we can
distribute
our programming tasks over several people or groups of people without
having
to have much communication between the people. We can tell somebody
that
we need a function that diagonalizes a matrix and we do not have to say
how we want it done. We will only specify the type of parameters to
pass
to the procedure.
For the same reason, we can easily use parts of other programs or
libraries
for
our purpose. In the ideal case we will just "link" those procedures
that
we will need to our program, without knowing exactly how they work. (Of
course
with knowing what they will do and how to call them)
- With procedures and functions the program becomes shorter, more
efficient
and more readible by avoiding repetitions of code and by organizing it
more logical.
Quick test:
To test your knowledge of what you have learned in this lesson, click here
for an on-line test.
Peter Stallinga. Universidade do Algarve, 17
março
2002