 |
Lecture 12: Modular Programming I:
Procedures
|
|
Until now we have only used the things that were supplied by PASCAL. We
have learned how to write loops (for, while-do, repeat-until), how to
have
input and output (readln, writeln), how to control the flow of the
program
(if-then, if-then-else, case-of), how to declare variables (var),
constants
(const), how to assign values to constants (:=) and how to calculate,
even
up to complicated Boolean algebra, but we have never invented anything
NEW. With Procedures and Functions we can do exactly that.
We already met a couple of procedures which are standard in PASCAL,
namely Write, WriteLn, Read e ReadLn.
Now we will define our own procedures.
Procedures and Functions are small subprograms or modules of
the main program. Each of theses modules performs a certain task. This
helps to organize the program and make it more logic and can also
increase
the programming efficiency by avoiding repetitions of parts of the
program.
There are two type of modules
-
Modules which don't return anything. In PASCAL these are called Procedures.
Of these there are two subtypes
-
Procedures that accept parameters and
-
Procedures that don't accept parameters as input
-
Modules that are returning values. In PASCAL these are called Functions.
Again, there are two subtypes
-
Functions that accept parameters and
-
Functions that don't accept parameters as input
Procedures
Modules that are not returning any value are called Procedures.
They just do something and don't return anything. They can either
accept
parameters (and work with them) or do things without any input from
outside.
In any case, Procedures are like programs-within-programs. They have
-
A name. The same rules for identifiers applies to the names of
procedures.
See aula 5
-
Variables and constants. These have to be declared in the Procedure
-
a begin and end; combination indicating the start
and
end of the procedure. Note that this end is finished with a ;
-
The program code
A prototype of the declaration of a procedure:
Procedure ProcedureName;
Var <variable_list>;
Const <const_list>;
begin
instructions;
end;
|
The place to declare a procedure is inside the program (so
after
the program name declaration), but before the beginning of the
main
program, so before the first begin statement.
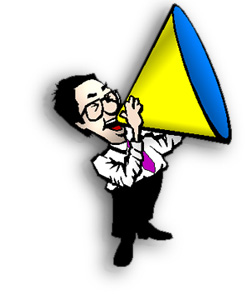 |
Calling
After declaring a new procedure, we can use it in the main program.
This
is called calling the procedure. We do
this
by writing the name of the procedure. As a complete example |
program code
PROGRAM WithProcedure;
Var x: real;
PROCEDURE Module1;
var y: real;
begin
WriteLn('Now I am entering
Procedure
Module1');
WriteLn('Give a value for y:');
ReadLn(y);
Writeln(y/3:0:3);
end;
begin
WriteLn('Starting the program');
Module1;
end.
|
output
Starting the program
Now I am entering Procedure Module1
Give a value for y:
12
4.000
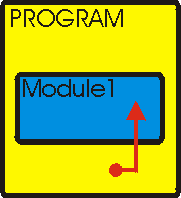
A program calling one of
its procedures
|
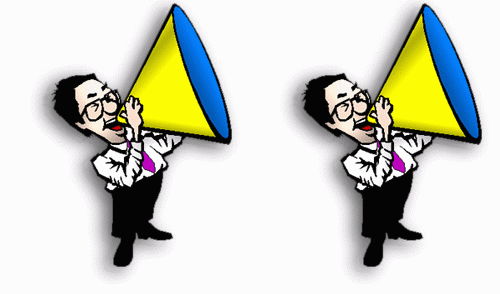 |
Procedures calling procedures
Procedures can also be called by other procedures or functions. Under
normal
circumstances, in PASCAL these procedures have to be declared after the
procedure to be called, though. Take a good look at the following
program
and the output it generates when run: |
program code
PROGRAM TwoProcedures;
Var x: real;
PROCEDURE Module1;
var y: real;
begin
WriteLn('Now I am entering
Procedure
Module1');
WriteLn('Give a value for y:');
ReadLn(y);
Writeln(y/3:0:3);
WriteLn('Leaving Module1');
end;
PROCEDURE Module2;
var z: real;
begin
WriteLn('Now I am entering
Procedure
Module2');
Module1;
WriteLn('Give a value for z:');
ReadLn(z);
Writeln(z*2:0:3);
WriteLn('Leaving Module2');
end;
begin
WriteLn('Now I am starting the
program');
Module2;
end.
|
output
Now I am starting the
program
Now I am entering Procedure
Module2
Now I am entering Procedure Module1
Give a value for y:
12
4.000
Leaving Module1
Give a value for z:
10
20.000
Leaving Module2
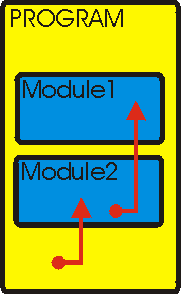
A program calling one of its procedures
which, in turn, is calling one other procedure
|
Use of variables
Yes: Procedures can
make
use of the variables of the main program, but not the other way around:
No: The main
program
cannot make use of the variables of the procedures. Think of the procedure
as a a box with one-way-mirror windows. From inside you can look
outside,
but from outside you do not see what is going on inside.
No: Procedures
cannot
use variables of the other procedures, for the same reason as why the
main
program cannot see the variables of the procedures. They can see the
other
procedures, but cannot look inside.
See the example program below. All the instructions in red
bold italics are forbidden.
program code
PROGRAM
ProcedureVariables;
Var x: real;
PROCEDURE Module1;
var y: real;
begin
WriteLn(x);
WriteLn(y);
WriteLn(z);
(*
this is not allowed *)
end;
PROCEDURE Module2;
var z: real;
begin
WriteLn(x);
WriteLn(y);
(*
this is not allowed *)
WriteLn(z);
end;
begin
WriteLn(x);
WriteLn(y);
(*
this is not allowed *)
WriteLn(z); (*
this is not allowed *)
end.
|
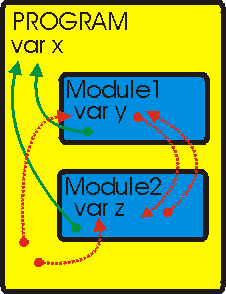
forbidden (red dashed arrows) and
allowed (green solid arrows)
variable use.
|
Later we will take a look at these things in more detail.
Nesting
In most programming languages Procedures can be nested. We can write
procedures within procedures within procedure within ....
Take a look at the program example below. The procedure within the
procedure is shown in bold. Just
like
the variables of the procedure Module1, the sub-procedure Module2 is
NOT
visible outside the Module1.
program code
PROGRAM
NestedProcedures;
Var x: real;
PROCEDURE Module1;
var y: real;
PROCEDURE Module2;
var z: real;
begin
WriteLn('Now I am
entering
Procedure Module2');
WriteLn('Give a
value
for z:');
ReadLn(z);
Writeln(z*2:0:3);
WriteLn('Leaving
Module2');
end;
begin
WriteLn('Now I am entering
Procedure
Module1');
WriteLn('Give a value for y:');
ReadLn(y);
Writeln(y/3:0:3);
Module2;
WriteLn('Leaving Module1');
end;
begin
WriteLn('Now I am starting the
program');
Module1;
(* cannot use
variables
or procedures *)
(* of Module1
here:
*)
(* cannot call
Module2
here *)
(* cannot use
variable
y or z here *)
end.
|
output
Now I am starting the
program
Now I am entering Procedure
Module1
Give a value for y:
12
4.000
Now I am entering Procedure Module2
Give a value for z:
10
20.000
Leaving Module2
Leaving Module1
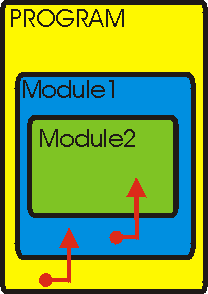
|
Quick test:
To test your knowledge of what you have learned in this lesson, click here
for an on-line test.
Peter Stallinga. Universidade do Algarve, 15
março
2002