 |
Lecture 6: Assignment, scanf and calculations
|
|
Assignment
In the previous lecture (aula 5) we have seen how
we can define variables. Now we will take a look at how the value of a
variable can be assigned a value
Giving a new value to a variable is called assignment. In C this
is done with the operator
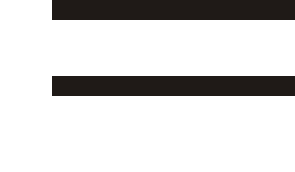
On the left side op this operator we put the variable, on the right side
we put the new value or expression that will produce a value of the
same type as the variable. Examples:
a = 3.0;
b = 3.0*a;
c = cos(t);
wrong (assuming a is of type float):
1.0 = a;
a = TRUE;
a = 1;
In reality, for C the last example was correct. C knows what we want
and helps us by converting the integer 1 to the real 1.0. In any
case, it is bad practice to mix floats with ints and we'd better write:
a
= 1.0;
The symbol = should be distinguished from the
normal mathematical symbol =. At this stage, it is interesting to make
a comparison between the mathematical symbol = and the assigment symbol
in programming languages (= in C). As an example take the following mathematical
equation
a = a + 1
This, as we all know, does not have a solution for a. (In comparison,
another example: a = a2 -
2, has a solution, namely a = 2).
In programming languages, however, we should read the equation differently:
a = a + 1;
means
(the new value of a) (will be)
(the old value of a) (plus 1)
Or, in other words: first the value of a is loaded from memory,
then 1 is added to it, and finally the result is put back in memory. This
is fundamentally different from the mathematical equation. In most other
modern languages the symbol = is used, which is confusing, especially
for the beginning programmer. That is why the student is advised to pronounce
the assigment symbol as 'becomes' or 'will be' instead of 'is' or 'equals'.
The symbol = should also be distinguished from the comparison symbol
=. For example a=b ? Later, we will learn how to make comparisons
(in the lecture on "if .. then" and "boolean algebra"), for which we will
use the == symbol.
To summarize, the = symbol is
NOT part of a comparison ("a is equal to b?")
NOT part of an equation ("a2 = 2a -1")
it IS an assignment ("a takes the value of b") |
Example
The following shows an example of a program using assignment statements
and the use of variables and constants. The right side shows the value
of the variables after each line
|
value of a
after executing the line
|
value of b
after executing the line
|
main()
{
float a;
float b;
float c = 4.3;
a = 1.0;
b = c;
a = a + b + c;
} |
undefined
1.0
1.0
9.6
|
undefined
undefined
4.3
4.3
|
Formatted input: scanf
In
the previous lectures we have learned how to show the results of our calculations
on the screen. With printf we can make our program have output.
In many cases, we would also like our program to have
input. The
user enters his name, or enters numbers that our program has to process.
Or even more simple, we want that the users can control the program. For
example, we want that the user can stop the program with a simple stroke
of the escape key.
C: With scanf we can get information from the keyboard. They
can read values and characters from the keyboard and directly store them
into specified variables. The general form the instructions take is
scanf("format decription(s)", &var1, &var2,
...);
|
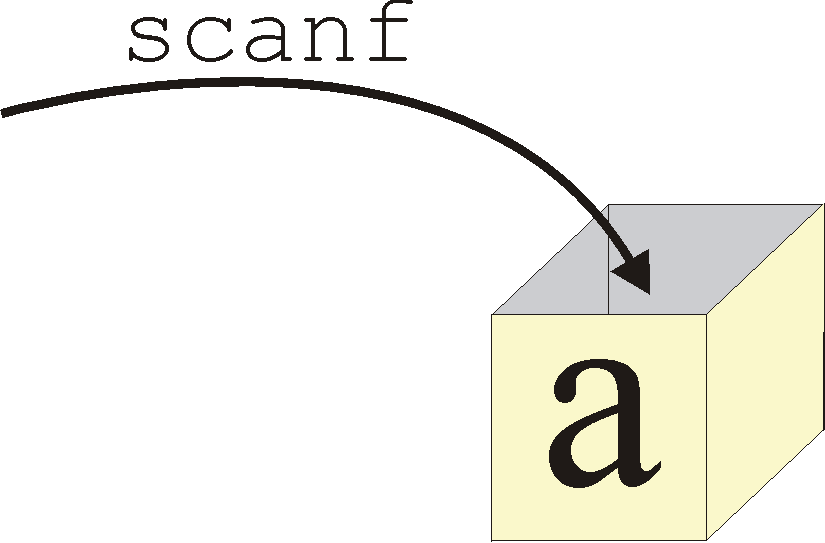 |
Note the symbol &. This means "the address of ..." We have
to give the address of the variable(s) to the function scanf.
As we will see later, giving the address of a variable is equal to "passing
by reference" and this allows for changes of the value of the variable
by the function. For the moment it suffices to say that we have to give
the address of the variables in scanf. In the analogy of the boxes
we could see it as giving the box to the function who then fills it with
a value.
The format descriptions are the same as for the output instruction printf.
For example %d for int's, etc. Note that the variables to be read
do not have to be of the same type.
As an example
// lines starting with these // are
cooment
// always when we use input or output
// we have to include the standard-I/O
library:
#include <stdio.h>
main()
{
// don't forget to declare the variables
we will use
int n1, n2: integer;
printf("Please enter two
numbers separated by a space\n");
scanf("%d", &n1);
scanf("%d", &n2);
printf("n1 = %d
n2 = %d", n1, n2);
}
When run, the program will display the message
Please, enter two numbers separated
by a space
The user is expected to type the two numbers
128 31<return>
(note the conventions we will use in this text: things the user will
enter will appear in green italics,
and <return> signifies
pressing the return key).
after which the program shows
n1 = 128 n2 = 31 |
Operations, operators and operands
After learning how to assign values above, we can now take a look how to
perform calculations with our programs. The most basic operations are adding,
subtracting dividing and multiplying:
operator
|
operation
|
+
|
addition
|
-
|
subtraction
|
*
|
multiplication
|
/
|
division
|
These four operators, when used for calculations, need two operands
and are called binary operators. In C one is placed on the left side and
one on the right side. As examples:
correct
|
wrong
|
3 * a
|
* a
|
a + b
|
3 a +
|
These expressions will result in values that can be assigned to variables
as we have seen above. As an example:
c = 3 * a;
Note again, on the left side of the assignment symbol = we have a variable
and on the left side we put our expression resulting in a new value for
the variable.
In C There also exists unary operators which need only one operand:
operator |
operation |
++
|
increment |
--
|
decrement |
!
|
negate (boolean algebra) |
~
|
invert all bits of this int |
-
|
negate number |
&
|
address of .. |
For example
i++;
--k;
Increases the value of i and decreases the value of k by 1 respectively.
The operator can be placed before or after the operator. The difference
is the moment the variable is changing value, before execution of the rest
of the instruction (++i) or after (i++). For example
i = 1;
j = i++;
at the end
i is equal to 2
j is equal to 1 |
i = 1;
j = ++i;
at the end
i is equal to 2
j is equal to 2 |
The ! and ~ operators we will see later in the lecture on Boolean algebra.
The get-address operator & will be discussed in the lecture
on pointers.
The unary - operator returns the negated number (floating point or
integer).
Combinations of calculation and assignment.
C has thte peculiar and confusing possibility to use a calculation and
assigment at the same time. For example:
c += a;
which is equivalent to
c = c + a;
Any binary operator can be used in this form (*=, /=,
+=,
-=).
This is confusing and as such should be avoided. On the other hand,
it can prevent errors. For example:
matrixelements[i+2*k-offset][n+m-f] = matrixelements[i+2*k-offset][n+m+f]
+ matrixelements[i+2*l+offset][n+m-f]
It is easy to make a typing mistake here. As a matter of fact, there
is a mistake in there. Did you spot it?.
Simpler and more readable would be:
matrixelements[i+2*k-offset][n+m-f] += matrixelements[i+2*l+offset][n+m-f]
Integer Math
The operators shown in the previous section are used for floating
point calculations. For integer calculations (used for types like byte,
word, integer, longint, etc), the division operator works a little different.
Imagine the calculation of, for example, 7/3. As we have learned in primary
school, this is equal to 2 with a remainder of 1 to be divided
by 3:
7
|
|
|
1
|
------
|
=
|
2 +
|
------
|
3
|
|
|
3
|
In C exist two operators / and % that reproduce these results. Example:
expression
|
result
|
7 / 3
|
2
|
7 % 3
|
1
|
These replace the floating-point operator /. The other three operators
(*, +, -) are the same for integer numbers.
Priority
In case there is more than one operator in an expression, the normal rules
of mathematics apply as to which one is evaluated first. The multiplication
and division operators have higher precedence. So, when we write
a = 1 + 3 * 2;
The result will be 7.
If we want to change the order at which the operators in the expression
are evaluated, we can always place parenthesis ( and ). So, for example
a = (1 + 3) * 2;
will result in 8. Putting parenthesis never hurts!
a = (1 + 3) - (4 + 5);
Examples
main()
/* an example floating point calculation
*/
{
double x, y, sum, diff, divis;
printf("Give the value of the first
variable x:\n");
scanf("%f", &x);
printf("Give the value of the second
variable y:\n");
scanf("%f", &y);
sum = x + y;
printf("The sum of %f and %f is %f\n",
x, y, sum);
diff = x - y;
printf("The difference between %f
and %f is %f\n", x, y, diff);
divis = x / y;
printf("%f divided by %f is %f\n",
x, y, divis);
}
will produce, when running:
Give the value of the first variable x:
3.4
Give the value of the second variable y:
1.8
The sum of 3.4000 and 1.8000 is 5.2000
The difference between 3.4000 and 1.8000
is 1.6000
3.4000 divided by 1.8000 is 1.8889
main()
/* an example integer calculation */
/* note the different format specifiers
in printf and scanf */
{
int x, y, sum, diff, divis, divr;
printf("Give the value of the first
variable x:\n");
scanf("%d", &x);
printf("Give the value of the second
variable y:\n");
scanf("%d", &y);
sum = x + y;
printf("The sum of %d and %d is %d\n",
x, y, sum);
diff = x - y;
printf("The difference between %d
and %d is %d\n", x, y, diff);
divis = x / y;
divr = x % y;
printf("%d divided by %d is %d plus
%d/%d\n", x, y, divis, divr, y);
}
will produce, when running:
Give the value of the first variable x:
13
Give the value of the second variable y:
5
The sum of 13 and 5 is 18
The difference between 13 and 5 is 8
13 divided by 5 is 2 plus 3/5
Quick test:
To test your knowledge of what you have learned in this lesson, click
here for an on-line test. Note that this is NOT the form the final
test takes!
Peter Stallinga. Universidade do Algarve, 10 October
2002