 |
Lecture 11: Modular Programming I:
Functions
|
|
Until now we have only used the things that were supplied by C. We have
learned how to write loops (for, while, do-while),
how to have input and output (scanf, and printf),
how
to control the flow of the program (if, if-else, switch),
how to declare variables, how to assign values to constants (=) and how
to calculate, even up to complicated Boolean algebra, but we have never
invented anything NEW. With Functions we can do exactly that.
We already met a couple of functions which are standard in C, namely
printf,
e scanf. Now we will define our own functions.
Functions are small subprograms or modules of the main
program.
Each of theses modules performs a certain task. This helps to organize
the program and make it more logic and can also increase the
programming
efficiency by avoiding repetitions of parts of the program. Moreover,
functions
allow for easy copying of parts of the code for other programs.
input/output
Modules (functions) can have input and/or output. In this case,
input means accepting parameters, while output means returning a value.
Note that, regardless of the fact if the functions accepts input
parameters,
the function always has parenthesis, both in the declaration as in the
function call, as we will see later. This is to distinguish functions
from
variables.
examples |
without
input
|
with
input
|
without
output |
abort() |
srand() |
with
output |
rand() |
sqrt() |
abort() stops the program, rand() returns a random
value,
srand()
initializes the random-number generator, sqrt() returns the
square
root of the argument.
This is the main difference between functions we know from mathematics
and functions from C. In mathematics, every function has input (the
argument)
and output (the function value). For example
f(x) = x2 -1
with x the input and f(x) the output. While in
C, we can have functions with and without input and output. Therefore,
the more genaral name for functions is "procedures", "(sub)routines",
or
"modules".
Functions are like programs-within-programs. They
-
must have a name. The same rules for identifiers applies to the names
of
functions. See aula 5.
-
can have variables. These have to be declared in the function and are
only
accessible by the function.
-
must have a { and } combination indicating the
start
and end of the function.
-
(can) have instructions.
A prototype of the declaration of a function:
type
functionname(type
<parameters>)
{
type
<variables>;
instructions;
}
|
output (type, void, return)
The type of the value the function will return has to be
specified
at the declaration of the function. For example
int countnumber();
will mean that the function will return a value of the type int.
Somewhere in the function we must return a value to the
calling
program. We do this with the return statement
return (<value>);
for example
return (3);
When want that the function doesn't return anything, we can specify
this with the word void:
void showresult();
will not return anything. Therefore, functions of type void don't need
the return statement.
Note: in some compilers the type declaration of the value to return
is optional. In some compilers, the absence of the type declaration
signifies
that the function is of type int, while in others it
signifies
void.
To add to the confusion, omitting a return statement is in some
compilers
an error, while in others it is not.
Therefore: stick to the conventions of standard ANSI C above and write
compiler-independent programs!
main()
In fact, main is nothing more than a normal function, with
the
only difference that the program always starts with the first
instruction
of this function main. Otherwise it follows the same rules of functions
described above. Technically speaking, we either have to specify the
type
as void or return something at the end of our program.
void main()
{
...
} |
int main()
{
...
return (0);
} |
placement
The place to declare our own fucntions is before the function main.
If we declare them after the function main we will not be able to use
them
inside main because the compiler does not know them yet when it arrives
at compiling main. A function can only call other functions that have
been
declared (in some way) before.
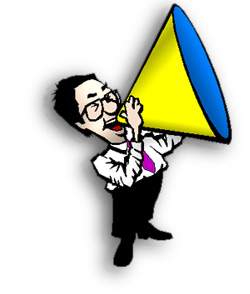 |
Calling
After declaring a new function, we can use it in the main program. This
is called calling the function. We do
this
by writing the name of the function. As a complete example of a program
with a single module (function) without input parameters or output
(void): |
program code
#include <stdio.h>
void module1()
{
int y;
printf("Now I am entering
Procedure
Module1\n");
printf("Give a value for y\n");
scanf("%d", &y);
printf("%d", 3*y);
// Type of function is void.
// No need to return anything.
}
void main()
{
// The program starts at the first
instruction
// of function main:
printf("Starting the program\n");
// now our function will be called:
module1();
printf("Ending the program\n");
}
|
output
Starting the program
Now I am entering Procedure Module1
Give a value for y:
4
12
Ending the program
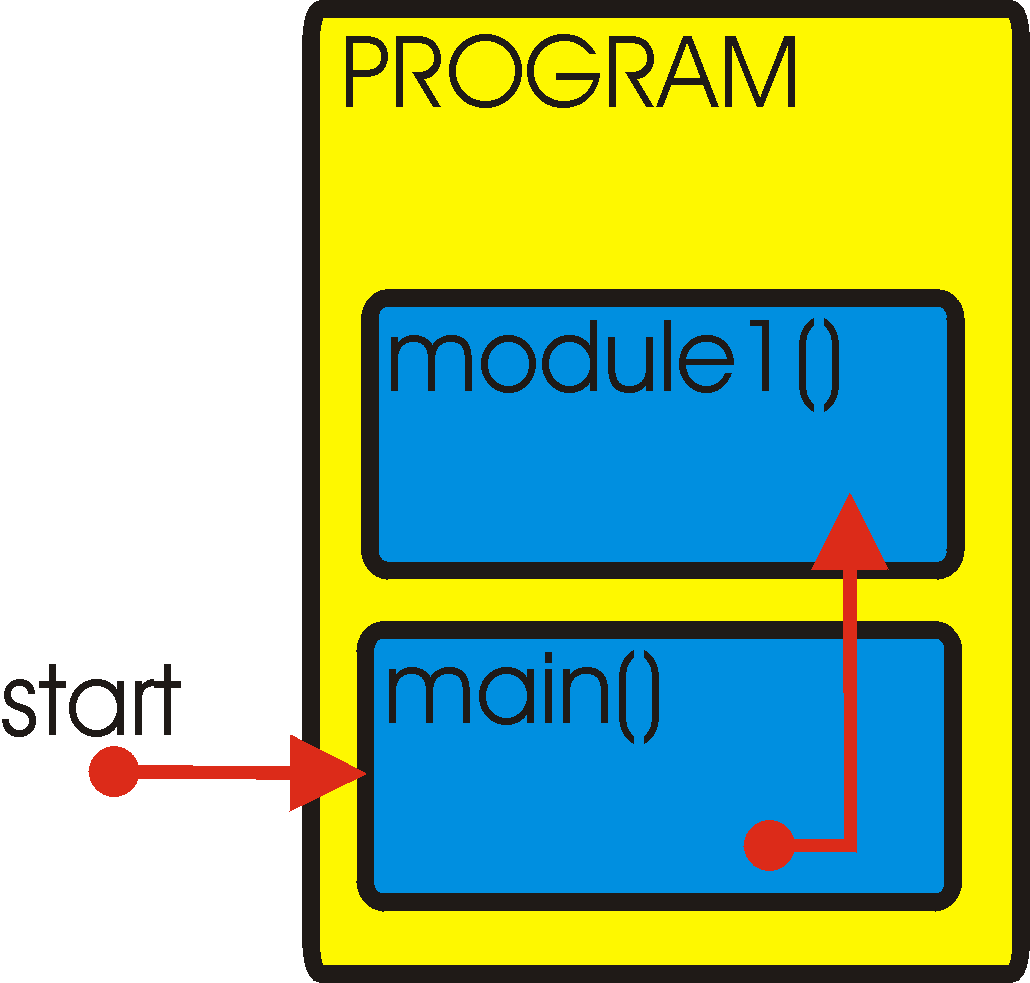
A program calling one of
its functions
|
As seen above, at the end of the function, the program continues with
the
instruction immediately after the function call. In this case, after module1()
has finished, it will execute printf("Ending
the program\n");
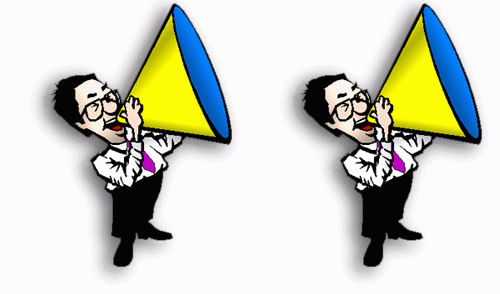 |
Functions calling functions
Functions can also be called by other procedures or functions. Under
normal
circumstances, in C these procedures have to be declared after the
procedure
to be called, though. Take a good look at the following program and the
output it generates when run: |
program code
# include
<stdio.h>
void module1()
{
printf("
inside
module 1\n");
printf(" Hello
World\n");
printf("
leaving
module 1\n");
}
void module2()
{
printf(" inside module
2\n");
printf(" calling module
1\n");
module1();
printf(" back in module
2\n");
printf(" leaving module
2\n");
}
void main()
{
printf("starting the program\n");
printf("calling module 2\n");
module2();
printf("back in main\n");
printf("ending program");
}
|
output
starting the program
calling module 2
inside module 2
calling module 1
inside module 1
Hello World
leaving module 1
back in module 2
leaving module 2
back in main
ending program
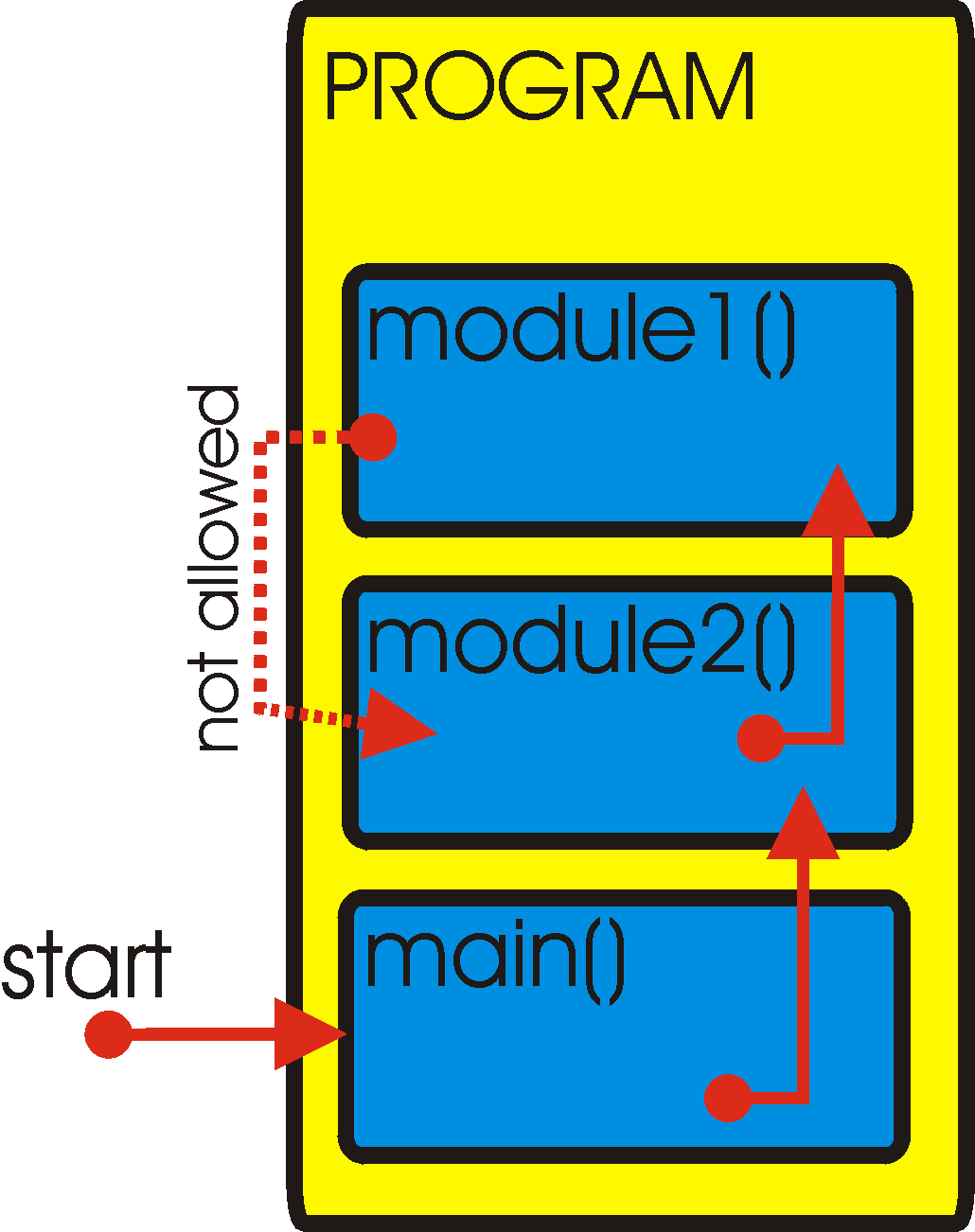
A program calling one of its functions
which, in turn, is calling one other function
|
Note that forward function calls, like in the above example a call
from
module1()
to module2(), are not allowed under normal circumstances.
main
can call module1() and module2(),
module2()
can call module1() and module1() can call nothing.
Quick test:
To test your knowledge of what you have learned in this lesson, click here
for an on-line test.
Peter Stallinga. Universidade do Algarve, 5
November
2002